While defining Bolina interfaces, and in order to make the developer’s life easier, we felt the need to scan the landscape of HTTP libraries. We started with Android HTTP Libraries and now moved to the other major player: iOS.
Here, I'll share with you what I have learned during this task, and start a brief discussion on the strengths and weaknesses of the most popular iOS HTTP libraries. You will also discover why I would choose using NSURLSession if I was about to start a brand new iOS application project.
Where to start?
Obviously, I had to start with URL Loading System, since it’s the iOS native library for HTTP handling and is surely one of the libraries that Bolina must support.
Moving toward the discovery of what else the developer's community was using I came through the projects of Alamofire Foundation: Alamofire and AFNetworking. Besides these two names, there were others that seemed to be under the community spotlight: Moya, SDWebImage, and Kingfisher.
The next step was to understand their usage among the community, and for that, I used the CocoaPods metrics API, which provides several metrics regarding the libraries usability. I also wanted to experience the “true pain” of using an iOS native networking library, so I created a demo project explaining how to fetch those values and prove these libraries fame was worth it.
The API has a lot of metrics available but I’ve decided to focus on those who could answer my question more rapidly: the total number of apps using, the total number of downloads and the number of stars in their GitHub repositories. The outcome was the following table:
Name |
Downloads |
Apps |
Stars on Github |
AFNetworking | 61983241 | 873085 | 30058 |
Alamofire | 42097177 | 662839 | 25398 |
SDWebImage | 45471101 | 626363 | 18622 |
Kingfisher | 8183631 | 105400 | 9798 |
Moya | 3395774 | 36629 | 7147 |
Let’s now and take a closer look into each one of them.
The URL Loading System
The URL Loading System is the native iOS set of tools and it provides access to resources (URL identified) using the HTTP protocol.
It seems to be the most used library among the community. I couldn’t find metrics to support this, but considering the sum of apps in the table above (approximately 2.3 million), knowing that there is a universe of 2 billions apps universe and assuming the most part of it makes use of networking services, that’s a pretty huge market share. The reasons behind it are clear: the easiness to maintain and the fact that all the other libraries are built on top of it.
All started with NSURLConnection, which wasn’t perfect - some people say that’s why Alamofire Foundation libraries came up. If you’re not aware, NSURLConnection is now deprecated so it is definitely not a good idea to use it. NSURLSession, which is basically a controller for network data transfer tasks, was presented as the alternative.
NSURLSession is supported in both iOS development languages, Objective-C and Swift - although, in the latest Swift 3 version, the NS prefix fell from all the Foundation types, giving place to the URLSession.
The URLSession provides asynchronous network calls, caching and even the implementation of custom protocols. This seems to be a perfect match if you’re looking for something to handle your HTTP requests and don’t want to invest much time refactoring or structuring code.
Pros
- Caching management
- HTTP prioritization
- HTTP/2 support
- Native library
- Support for RESTful API’s
- Good documentation
- Customizable protocols
Cons
- No explicit use of synchronized calls
- Low-level management at the socket layer
- Low-level management of insecure connections
- Need to test our network code (vs. trusting a widely tested lib)
AFNetworking and Alamofire
Alamofire and AFNetworking are both libraries developed by the Alamofire Software Foundation and its major focus is to simplify HTTP networking in iOS.
The eldest one, AFNetworking, is based on Objective-C and it was created to help developers overcome the pains of using NSURLConnection, introducing more features, such as the asynchronous load of images into the UI. It still has more users than Alamofire, but its usage was hardly questioned when a major security issue was spotted.
Alamofire is the Swift version of its big brother, with similar patterns, features, and purpose. It struggles to try to keep up with Swift programming language evolution, but still has a huge and solid base of fans.
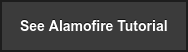
Pros
- Simple API
- Complete control of HTTP connections
- Very good documentation
- NSURLSession under the hood
- HTTP/2 support
- Supports both objective-c and swift languages
Cons
- Prior versions will demand code refactoring in order to update the lib version
- Some security issues were spotted
SDWebImage, Kingfisher
SDWebImage and Kingfisher are libraries totally focused on images downloads, cache, and presentation. Although this could be a limitation in many cases, they seem to be highly requested by developers. They both handle asynchronous memory and disk image caching with automatic cache expiration. The only setback is that some users report having experienced some memory warning issues with both libraries.
Contrary to Kingfisher, SDWebImage language is pure Objective-C which can help when choosing between both libraries.
Pros
- Simple API
- Swift support
- Good documentation
- UI Image load handlers
- NSURLSession under the hood
Cons
- Only handle images
- Only useful for downloads
Moya
Another commonly used library is Moya, although it isn’t exactly a network library. They claim to be a “Network abstraction layer” instead. The following image, borrowed from their documentation, is a very good explanation on that.
Moya library is written in Swift and it’s basically a wrapper around Alamofire library, which also can be seen as a wrapper around the native URL Loading System. If you’re looking for a way to encapsulate and abstract the network layer within your app, this is probably a solution to consider.
Pros
- Good quality documentation
- NSURLSession under the hood
- Architecture abstraction layer
Cons
- All Alamofire’s cons (once it’s built on top of it)
Wrapping up
There are a lot of things you should take into account when choosing among the iOS HTTP Libraries, and every little detail can make a difference. Generally speaking, I would go for the NSURLSession: it has everything we need with respect to tools and a very low probability of being deprecated soon. In addition, when it comes to Apple, I will always choose to avoid third-party libraries, not only for security reasons but also to avoid a lack of support and maintainability. You can read more about my experience with NSURLSession here.
It’s also my opinion that the other libraries aren’t game changers when compared to the native lib, of course, this is debatable (the debate exists and seems broad), but again, that’s my personal view on it.
On the other hand, if my app would fetch and present a large number of images, I would definitely use SDWebImage or Kingfish, but again: that would depend a lot of other aspects that we need to consider, such as the trade-off between performance and business logic.